Hi, guys I am back with another fantastic project in this project I created an Analog Clock Using HTML, CSS & JavaScript.
I provide my readers and followers with the complete source code for this project without any cost or subscription requirements. The code files are available for free download and use.
Analog Clock
An analog clock is a timekeeping device that displays the time using the position of rotating hands or pointers on a circular dial. It typically consists of a circular face divided into 12 hours, with each hour marked by numerals or indices.
The clock hands include an hour hand, a minute hand, and sometimes a second hand, all rotating around a central point. The hands point to the current time on the clock face, providing a visual representation of the hours, minutes, and sometimes seconds.
Analog clocks are often used in various places, such as homes, offices, and public spaces. They have a classic and traditional design and are appreciated for their simplicity and ease of reading.
Digital clocks, on the other hand, display time using numerical digits and are common in electronic devices like smartphones, computers, and digital watches.
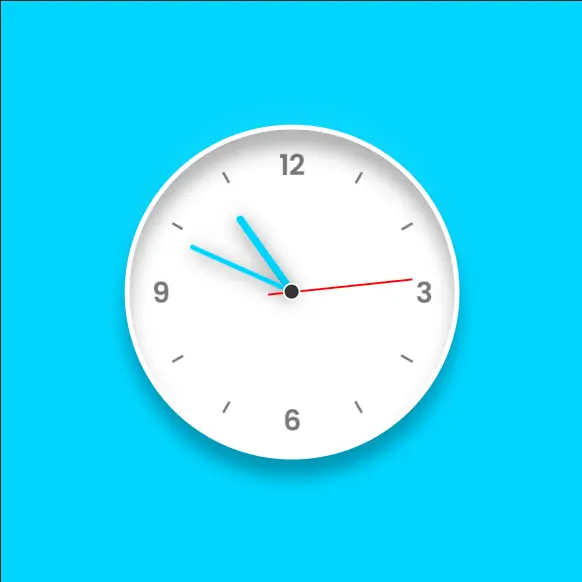
Analog Clock Using JavaScript
An analog clock created using JavaScript involves dynamically updating the rotation of clock hands based on the current time. Here’s a breakdown of the key components and the process:
1. HTML Structure:
- The clock is represented as a circular div (
#clock
) with a border to create the appearance of a clock face. - Inside the clock, there are three div elements (
#hourHand
,#minuteHand
, and#secondHand
) representing the hour, minute, and second hands, respectively.
2. CSS Styling:
- The clock is styled using CSS to define its appearance, such as size, border, and circular shape.
- Each clock hand (
#hourHand
,#minuteHand
, and#secondHand
) is styled with a background color and dimensions. - The
transform-origin
property is used to set the rotation pivot point for each hand at the bottom center (50% from the left, 100% from the top).
3. JavaScript Logic:
- The
updateClock
function is defined to calculate the rotation angle for each clock hand based on the current time. - The
Date
object is used to get the current hours, minutes, and seconds. - Conversion formulas are applied to convert hours, minutes, and seconds into degrees for rotation.
- The
transform
property in CSS is then updated for each clock hand to reflect the calculated rotation angles.
4. Time Update:
- The
setInterval
function is used to call theupdateClock
function every second (1000 milliseconds), ensuring that the clock hands update continuously. - The initial call to
updateClock
is made to set the initial positions of the clock hands based on the current time.
The HTML defines the structure, the CSS styles the clock and hands, and the JavaScript dynamically updates the rotation angles of the hands based on the current time, creating a functional analog clock.
About This Project
The Code Hunter team developed this project to assist students in learning coding and programming. If you’re interested in joining our team, please follow us on Instagram.
Created by | Ahmed Developer |
Languages | HTML, CSS & JS |
Source Code | Available Below |
Preview | Take Preview |
GitHub Link | Code Link |
How to Create a Analog Clock Using HTML, CSS, and JS
There are some steps to create this type of Analog Clock Using HTML, CSS, and JavaScript which are given below.
- The first step is to create one folder.
- Open this folder in Vs Code Editor and create three (3) files for HTML, CSS, and JavaScript.
- Make sure the HTML file extension is (.html), and the CSS file extension is (.css).
- After creating files, you link a CSS file with an HTML file with the help of this line code. (<link rel=”stylesheet” href=”style.css”>)
- The last step is copying and pasting the given code into your files.
Video Tutorial
If you want to learn more about this project please watch this video and also subscribe to this YouTube channel for more content like this. This video is provided by Online Tutorials.
Analog Clock Using HTML, CSS, and JavaScript – Source Code
HTML FILE
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> </head> <body> <div class="clock"> <div class="hour"></div> <div class="min"></div> <div class="sec"></div> </div> <div class="attribute"> Create by <a href="https://www.linkedin.com/in/ahmed-raza-developer/"> Ahmed Developer </a > Inspired by <a href="https://www.youtube.com/watch?v=weZFfrjF-k4 "> Click here </a > </div> <script src="script.js"></script> </body> </html>
CSS FILE
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;700&display=swap'); :root{ --clr-primary: rgba(0,213,255,1); --clr-secondary: #333; --clr-container-bg: #fff; --clr-btn-bg: rgb(0, 122, 209); --clr-btn-hover-bg: rgba(0,175,255,1); --clock-primary-shadow : -2px 2px 15px #333333b4; --clock-inset-shadow:0 -15px 15px rgba(255, 255, 255, 0.05),inset 0 -15px 15px rgba(255, 255, 255, 0.05), 0 15px 15px rgba(0, 0, 0, 0.3),inset 0 15px 15px rgba(0, 0, 0, 0.3); } *{ box-sizing: border-box; margin: 0; padding: 0; } body { font-family: sans-serif; min-height: 100vh; width: 100%; display: flex; align-items: center; justify-content: center; background-color: var(--clr-primary); font-family: 'poppins'; position: relative; } .clock { min-height: 18em; min-width: 18em; display: flex; justify-content: center; align-items: center; background-color: var(--clr-container-bg); background-image: url("https://imvpn22.github.io/analog-clock/clock.png"); background-position: center center; background-size: cover; border-radius: 50%; border: 4px solid var(--clr-container-bg); box-shadow: var(--clock-inset-shadow); transition: all ease 0.2s; } .clock:before { content: ""; height: 0.75rem; width: 0.75rem; background-color: var(--clr-secondary); border: 2px solid var(--clr-container-bg); position: absolute; border-radius: 50%; z-index: 1000; transition: all ease 0.2s; } .hour, .min, .sec { position: absolute; display: flex; justify-content: center; border-radius: 50%; } .hour { height: 10em; width: 10em; } .hour:before { content: ""; position: absolute; height: 50%; width: 6px; background-color: var(--clr-primary); border-radius: 6px; box-shadow: var(--clock-primary-shadow); } .min { height: 12em; width: 12em; } .min:before { content: ""; height: 50%; width: 4px; background-color: var(--clr-primary); border-radius: 4px; box-shadow: var(--clock-primary-shadow); } .sec { height: 13em; width: 13em; } .sec:before { content: ""; height: 60%; width: 2px; background-color: #f00; border-radius: 2px; } .attribute{ position: absolute; bottom: 10px; }
JavaScript FILE
const deg = 6; const hour = document.querySelector(".hour"); const min = document.querySelector(".min"); const sec = document.querySelector(".sec"); const setClock = () => { let day = new Date(); let hh = day.getHours() * 30; let mm = day.getMinutes() * deg; let ss = day.getSeconds() * deg; hour.style.transform = `rotateZ(${hh + mm / 12}deg)`; min.style.transform = `rotateZ(${mm}deg)`; sec.style.transform = `rotateZ(${ss}deg)`; }; // first time setClock(); // Update every 1000 ms setInterval(setClock, 1000);
You Might Like This:
- How To Build QR Code Generator in JavaScript – Complete Source Code Available
- Modern To-Do List App Using HTML, CSS, and JavaScript – Complete Source Code
- How to Create a Digital Clock Using JavaScript Complete Source Code
Final Words
Building an analog clock with HTML, CSS, and JavaScript is a fascinating blend of design and functionality. HTML structures the clock, CSS styles it, and JavaScript dynamically updates the hands based on the current time.
This tutorial not only provides technical insights but also emphasizes the collaborative nature of web development, showcasing the synergy between these three technologies. Creating an analog clock is a practical exercise that enhances both design and coding skills, offering a hands-on experience in front-end development.